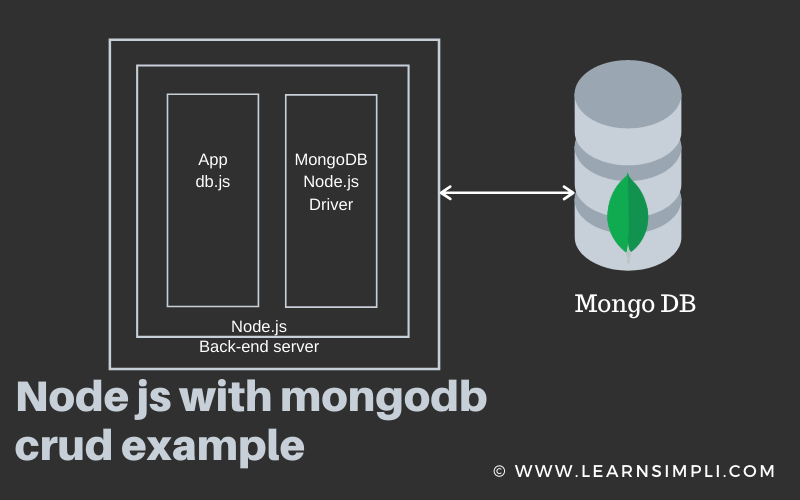
Node js with MongoDB crud example
Node js with MongoDB crud example, MongoDB comes with drivers those can be used with supported languages like Nodejs, C, C++, Java, PHP, and Python. This article is related to CRUD operations in Nodejs with MongoDB. To start developing an application, Nodejs with MongoDB driver you can follow the below step to step guidelines.
How to install MongoDB in the system?
Download the MongoDB package and install it in your system.
https://www.mongodb.com/download-center
If you have already installed then you can skip the step 1
How to install the MongoDB Driver in Nodejs?
Create your application directory
- mkdir mongodbDemo
- cd mongodbDemo
- Run the command
- npm init
In order to utilize the MongoDB database in Nodejs, you need to install the MongoDB driver by using the NPM.
- npm install mongodb –save
- Once installation is done you can set the path for mongodb
- mongod –dbpath=/data
How to Connect to MongoDB in Nodejs?
Create a new index.js file and add the following code.
const MongoClient = require('mongodb').MongoClient; const assert = require('assert'); // Connection URL const url = 'mongodb://localhost:27017'; // Database Name const dbName = 'companyDB'; // Use connect method to connect to the server MongoClient.connect(url, function(err, client) { assert.equal(null, err); console.log("Connected successfully to mongodb server"); const db = client.db(dbName); client.close(); }); Run the command node index.js // Connected successfully to mongodb server
How to Insert a Document in MongoDB with Nodejs?
Now we will create a database called companyDB, and we are gonna insert 5 employee records.
Put the below code in index.js (replace with previous code)
const MongoClient = require('mongodb').MongoClient; const assert = require('assert'); // Connection URL const url = 'mongodb://localhost:27017'; // Database Name const dbName = 'companyDB'; // Use connect method to connect to the server MongoClient.connect(url, function(err, client) { assert.equal(null, err); console.log("Connected successfully to Mongodb server"); const db = client.db(dbName); insertDocuments(db, function() { client.close(); }); }); const insertDocuments = function(db, callback) { // Get the documents collection const collection = db.collection('emplyees'); // Insert some documents collection.insertMany([ {id : 1, "Name":"Employee 1"}, {id : 2, "Name":"Employee 2"}, {id : 3, "Name":"Employee 3"}, {id : 4, "Name":"Employee 4"}, {id : 5, "Name":"Employee 5"}, ], function(err, result) { assert.equal(err, null); assert.equal(5, result.result.n); assert.equal(5, result.ops.length); console.log("Inserted 5 employee details into the collection"); callback(result); }); } Run the command node index.js // Connected successfully to MongoDB server // Inserted 5 employee details into the collection
How to search or find documents in MongoDB with Nodejs?
Now we will search a record that matches the employee names
Put the below code in index.js (replace with previous code)
const MongoClient = require('mongodb').MongoClient; const assert = require('assert'); // Connection URL const url = 'mongodb://localhost:27017'; // Database Name const dbName = 'companyDB'; // Use connect method to connect to the server MongoClient.connect(url, function(err, client) { assert.equal(null, err); console.log("Connected successfully to Mongodb server"); const db = client.db(dbName); findDocuments(db, function() { client.close(); }); }); const findDocuments = function(db, callback) { // Get the documents collection const collection = db.collection('emplyees'); // Find some documents collection.find({'Name': "Employee 1"}).toArray(function(err, docs) { assert.equal(err, null); console.log("Found the following records"); console.log(docs) callback(docs); }); } // Connected successfully to Mongodb server // Found the following records // [ // { _id: 5d7f5814dfb3bf14700b68d7, id: 1, Name: 'Employee 1' }, // { _id: 5d7f5916433e6004380ae56b, id: 1, Name: 'Employee 1' } // ]
How to update documents in MongoDB with Nodejs?
Now we will update a record that matches the employee id
Put the below code in index.js (replace with previous code)
const MongoClient = require('mongodb').MongoClient; const assert = require('assert'); // Connection URL const url = 'mongodb://localhost:27017'; // Database Name const dbName = 'companyDB'; // Use connect method to connect to the server MongoClient.connect(url, function(err, client) { assert.equal(null, err); console.log("Connected successfully to mongodb server"); const db = client.db(dbName); updateDocument(db, function() { client.close(); }); }); const updateDocument = function(db, callback) { // Get the documents collection const collection = db.collection('emplyees'); // Update document where a is 2, set b equal to 1 collection.updateOne({ "Name" : "Employee 1" } , { $set: { "Name" : "Employee 1 updated " } }, function(err, result) { assert.equal(err, null); assert.equal(1, result.result.n); console.log("Updated the document with the field a equal to employee 1"); callback(result); }); } // Connected successfully to mongodb server // Updated the document with the field a equal to employee 1
How to delete documents in MongoDB with Nodejs?
Now we will delete a record that matches the employee id
Put the below code in index.js (replace with previous code)
const MongoClient = require('mongodb').MongoClient; const assert = require('assert'); // Connection URL const url = 'mongodb://localhost:27017'; // Database Name const dbName = 'companyDB'; // Use connect method to connect to the server MongoClient.connect(url, function(err, client) { assert.equal(null, err); console.log("Connected successfully to server"); const db = client.db(dbName); removeDocument(db, function() { client.close(); }); }); const removeDocument = function(db, callback) { // Get the documents collection const collection = db.collection('emplyees'); // Delete document where a is 3 collection.deleteOne({ "Name":"Employee 1" }, function(err, result) { assert.equal(err, null); assert.equal(1, result.result.n); console.log("Removed the document with the field a equal to employee 1"); callback(result); }); } // Connected successfully to server // Removed the document with the field a equal to employee 1
Also, read Mongodb vs mysql which is better?
One thought on “Node js with mongodb crud example”
Comments are closed.