Control flow statements in python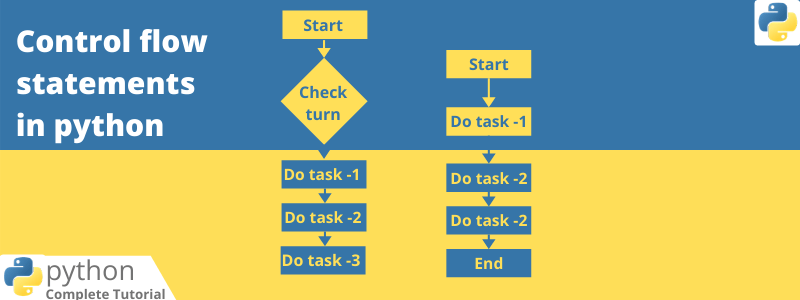
In this chapter, you will learn about control flow statements in python. In python there are various methods that can be used for executing only a certain block of statements and grouping the set of statements based on the condition. The statement is a set of instruction which performs some kind of operations. Now let’s list the available control flow in the python.
When you compare the python with other languages, the python is a bit different in syntax. Let’s list the points which make the python different from other languages
- All control flow statements use the colons
- After the very first line of the control flow statement, there will be an indentation
if-else statement:
The if-else statement starts with if and ends with else. The if-else statement can be used to check whether some condition is true or not.
if 3 > 2: print('Number is greater') else: print('Number is not greater') # output # Number is greater
You can use the more than one if condition, let’s write an example for checking the multiple if conditions
if 3 > 3: print('Number is greater') elif 3==3: print('Number is equal') else: print('Number is not greater') # output # Number is greater # Number is equal
For loops:
Every language provides for loops statements that can iterate through an object like string, list, and object. You can write any kind of if-else condition inside the loop. Let’s see the syntax and write an example for loop
for loop using the lists:
When you use a list for a loop, it behaves the same like which we use in the other languages. Let’s write an example of the same.
studentName = ['John','Stark','Mickel'] for name in studentName: print(name) # output # John # Stark # Mickel
for loop using the tuples:
When we use tuples in the for loop, the tuples have some advantages. Now we will check whether the student is failed in the exam or pass, let’s write the code snippet for the same.
studentMarks = [('John',35,30),('Stark',35,55),('Mickel',35,55),('Clark',35,65)] for studentName, minMarks, obtainedMarks in studentMarks: if obtainedMarks >= minMarks: print(studentName +' has been passed...') else: print(studentName+' has been failed...') # output # John has been failed... # Stark has been passed... # Mickel has been passed... # Clark has been passed...
for loop using the dictionary:
studentMarks = {'k1':1,'k2':2,'k3':3} for key, value in studentMarks.items(): print('************ Key ************') print(key) print('************* Value **********') print(value) # output # ************ Key ************ # k3 # ************* Value ********** # 3 # ************ Key ************ # k2 # ************* Value ********** # 2 # ************ Key ************ # k1 # ************* Value ********** # 1
While loops:
While loop will keep executing a block of code while some condition remains true. For example, while the battery is not full, keep charging the battery
batteryLevel = 0 while batteryLevel <= 100: print(f'Battery level {batteryLevel}') batteryLevel = batteryLevel + 10 # output # Battery level 0 # Battery level 10 # Battery level 20 # Battery level 30 # Battery level 40 # Battery level 50 # Battery level 60 # Battery level 70 # Battery level 80 # Battery level 90 # Battery level 100
Now we will look into the following keywords
break:
Breaks out of the current closest enclosing loop
continue:
Goes to the top of the closest enclosing loop
pass:
Does nothing at all
break:
Now we will look into the break, and how it can be used in the looping. The break keyword will exit from the loop
studentName = 'John' for letter in studentName: if letter == 'h': break print(letter) # output # J # o
continue:
Now we will look into the continue keyword and how it can be used. Let’s write an example, as we can see in the below code snippet output, when a condition meets then it will not execute the remaining lines in the for loop block, instead it jumps to the top.
studentName = 'John' for letter in studentName: if letter == 'h': continue print(letter) # output # J # o # n
pass:
Now we will learn how to pass can be used,
studentName = ['John','Stark','Mickel'] for name in studentName: # comment print('For loop is been finished...') # output # IndentationError: expected an indented block
To fix the above error we can use the pass keyword, the above code snippet is generating an error as there is a comment line after the statement.
studentName = ['John','Stark','Mickel'] for name in studentName: # comment pass print('For loop is been finished...') # output # For loop is been finished...
One thought on “Control flow statements in python”
Comments are closed.