Data types in python with an example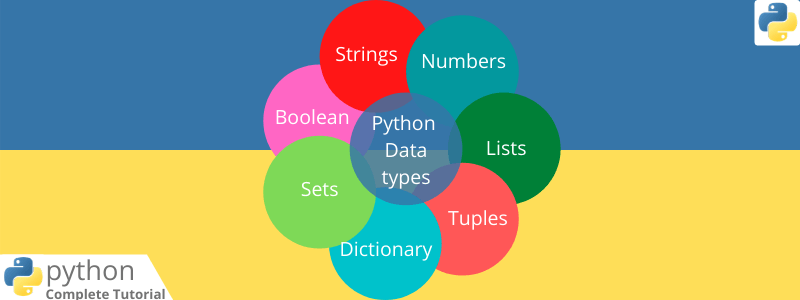
In this chapter, you will learn about what are the data types available in the python programming language. The python has inbuilt data types that are grouped into the following categories.
Text type
In the text type data group you perform operations related to the string and a good number of methods are available to perform operations on a string. Syntax
str
Numeric type
In the Numeric type data group, you can perform operations related to integers, float, and complex numbers. Syntax
int
float
complex
Sequence type
The sequence type category support’s for storing order and unordered list and also a good number of methods to perform operations on the list, we will discuss the same with an example in the same chapter.
Mapping type
The mapping type helps to define objects like a dictionary. Syntax
dict
Set type
The set type group can create the set and frozenset data types we will look more into in the same chapter below.
Bool type
In this group, we can create Boolean data types like true or false Syntax
bool
Binary type
In the binary type category, you can create the binary data types like bytes and bytes arrays and memory view.
“In the python, the variable type will be created when you assign a value to the variable”
Now we will define each data type and write an example for all the data types in python. Create a file
python script/data-types-example-in-python.py
Number:
In the number data type, you can define the various variables like number float and complex. Let’s write an example for implementing the same. Now let’s check what data types have been created by the python by using the method type()
age =10 score= 90.5 personId= 2j print(type(age)) print(type(score)) print(type (personId)) # Output # <type 'int'> # <type 'float'> # <type 'complex'>
Now let’s see each supported data types in Number.
Int:
The int stands for integer and it can hold the whole number both positive and negative numbers. The length of a number depends on the memory of your operating system basically there is no limit on the length of the number in python. Note that the integer data type doesn’t contain the decimal point
pesrionAge=30 print(pesrionAge) print(type(pesrionAge)) # Output # 30 # <type 'int'>
Float:
The float data type can hold the integer number with a decimal point.
personWeight = 55.55 personScore = float(90.5) print(personWeight) print(personScore) print(type(personWeight)) print(type(personScore)) # output # 55.55 # 90.5 # <type 'float'> # <type 'float'>
Complex:
Let’s create a complex type
personId = complex(1j) print(personId) print(type(personId)) # output # 1j # <type 'complex'>
Strings:
Strings can hold the sequence of characters, strings can be defined or enclosed within the single quotes or double-quotes. And backslash \ can be used to escape quotes inside the string. Let’s write an example for defining the string.
studentName = 'John\'s' motherName = str('Marry') schoolName = '''International primary school ''' print(studentName) print(motherName) print(schoolName) print(type(studentName)) print(type(motherName)) print(type(schoolName)) # output # John's # Marry # International # primary # school # <type 'str'> # <type 'str'> # <type 'str'>
Lists:
The lists data type is similar to the javascript array. The list can be defined by using the square brackets and values can be grouped by separating with the comma. The following points can help you to understand the lists in a better way
- Lists can be indexed and sliced
- Lists can hold items of different types or similar types
- Lists also support operations like concatenation
- Lists are a mutable
studentMarks = [50,60,35,70,87,45] studentSubjects = x = list(('Maths', 'English', 'Kannad')) print(studentMarks) print(studentSubjects) print(type(studentMarks)) print(type(studentSubjects)) # output # [50, 60, 35, 70, 87, 45] # ['Maths', 'English', 'Kannad'] # <type 'list'> # <type 'list'>
Boolean:
As similar to all other programming languages, python has the Boolean type which holds the True or False. The first letter of the True and False is the capital.
isAdmin = True isSuperAdmin = False print(isAdmin) print(isSuperAdmin) print(type(isAdmin)) print(type(isSuperAdmin)) # output # True # False # <type 'bool'> # <type 'bool'>
Tuple:
A tuple can hold the ordered and unchangeable collection. Tuple can be defined by using the round brackets.
studentMarks = (50,60,35,70,87,45) studentSubjects = x = tuple(('Maths', 'English', 'Kannad')) print(studentMarks) print(studentSubjects) print(type(studentMarks)) print(type(studentSubjects)) # output # (50, 60, 35, 70, 87, 45) # ('Maths', 'English', 'Kannad') # <type 'tuple'> # <type 'tuple'>
Set:
A set object is an unordered collection of distinct hashable objects. Common uses include membership testing, removing duplicates from a sequence, and computing mathematical operations such as intersection, union, difference, and symmetric difference
studentSubjects = x = {'Maths', 'English', 'Kannad'} print(studentSubjects) print(type(studentSubjects)) # output # set(['Maths', 'Kannad', 'English']) # <type 'set'>
Dict:
A mapping object maps hashable values to arbitrary objects. Mappings are mutable objects. A dictionary’s keys are almost arbitrary values. Values that are not hashable, that is, values containing lists, dictionaries or other mutable types may not be used as keys. Dictionaries can be created by placing a comma-separated list of key: value pairs within braces.
student = { "name": "John", "score": 90, "school": "International school" } print(student) print(type(student)) # output # {'school': 'International school', 'score': 90, 'name': 'John'} # <type 'dict'>
3 thoughts on “Data types in python with an example”
Comments are closed.