Methods and functions in python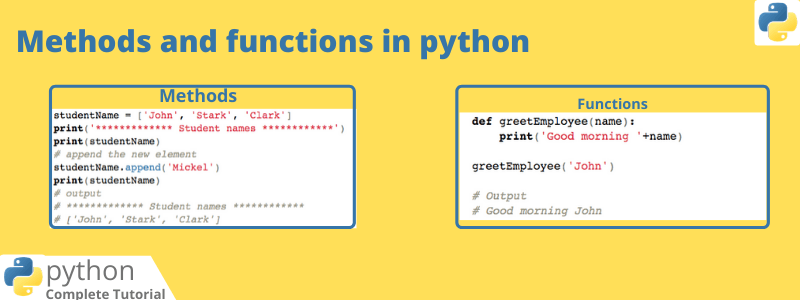
In this chapter, you will learn about Methods and functions in python. We will look into the methods first.
What are the methods in python?
- Methods are simply functions which are built into objects
- Methods are dependent on classes, which means methods can be created with the help of class
- Python provides a lot of inbuilt methods like Len list methods pop append etc
- Methods can return a value or entity
Let’s write an example for using the inbuilt methods
studentName = ['John', 'Stark', 'Clark'] print('************* Student names ************') print(studentName) # append the new element studentName.append('Mickel') print(studentName) # output # ************* Student names ************ # ['John', 'Stark', 'Clark']
What are the functions in python?
- Functions help in creating a block of code that can be easily executed many times without needing to rewrite the entire block of code
- Functions can be defined with the keyword def followed by a function name and followed by open and close brackets
- Functions can receive arguments or parameters explicitly
- Functions are independent of classes, which means you can create functions without classes
- In functions, you can use the return key to assign the result of the function to a new variable and then return it
Let’s write an example of how to create a function and call it
def greetEmployee(name): print('Good morning '+name) greetEmployee('John') # Output # Good morning John
Now the above function is just printing the message but it’s not returning anything. You can use the return keyword to return the result of the function and you can use it later whenever you need it.
def greetEmployee(name=''): return 'Good morning '+name message = greetEmployee('John') print(message) # Output # Good morning John
As we can see in the above code, the function now returning the message instead of printing.
How to send the dynamic parameters with the key?
Now we have passed the single parameter to the function, what about if we need to send the dynamic arguments, for sending the dynamic parameters we can use the *args. Let’s write an example for the same
def add_numbers(*params): return sum(params) totalSum = add_numbers(1,2,3,4) print(totalSum) # Output # 10 totalSum = add_numbers(6,5,7,8,2,2) print(totalSum) # Output # 30
How to send the dynamic parameters with the key?
You can the **params to send the dynamic parameters with the key. Let’s write an example of the same.
def greet_employee(**params): if 'name' in params: message = 'Hi good morning '+params['name'] else: message = 'Hi good morning...' return message getMessage = greet_employee(name = 'John') print(getMessage) # output # Hi good morning John getMessage2 = greet_employee(email = 'John@email.com') print(getMessage2) # output # Hi good morning...
One thought on “Methods and functions in python”
Comments are closed.